A typical operation in a range of scientific, mathematical, and programming applications is to normalize a vector or a matrix. Today, we are going to learn how to normalize NumPy array to a unit vector. We will learn what normalization is and how to compute it in Python in this tutorial.
Introduction To Numpy Array
Vectors or matrices of numbers are most commonly represented using NumPy arrays. A vector is represented as a 1-D array, and a matrix is defined by a 2-D array (where each row/column is a vector).
Vectors are spatial entities. There is a magnitude and a direction to them. The transformation of a vector obtained by executing specific mathematical operations on it is known as normalization. We calculate a value called the “norm” of a vector to do normalizing.
Numpy Norm
For functions related to linear algebra, NumPy has a dedicated submodule named linalg.
This submodule contains Python functions for performing typical linear algebraic operations such as vector products, solving equations, eigenvalues calculation, etc.
The following function used for finding norms of vectors and matrices – numpy.linalg.norm().
Why Do We Need Norms?
Normalization is a fairly popular procedure in a number of applications, as stated in the introduction.
One significant application of norm is to convert a given vector into a unit-length vector, i.e., make its magnitude as 1 while keeping the direction.
In many machine learning methods, normalisation is also a key pre-processing step.
How To Normalize NumPy Array To A Unit Vector
A vector is a quantity that has both, magnitude and direction. A unit vector is a vector with a magnitude of one. The numpy.linalg.norm() function can be used to normalize a vector to a corresponding unit vector. There are many functions in the numpy.linalg package that are relevant in linear algebra. To determine the norm of a vector, we can utilize the norm() function in numpy.linalg. We can retrieve the vector’s unit vector by dividing it by its norm.
There Are Multiple Ways To Convert A NumPy Arrow To A Unit Vector
- Get unit vector using an inbuilt function:
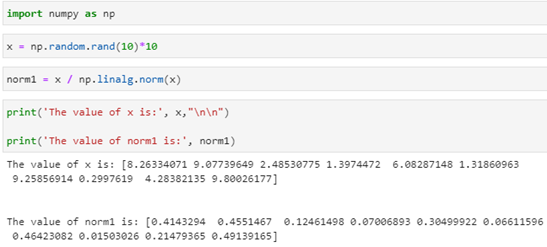
import numpy as np
x = np.random.rand(10)*10
norm1 = x / np.linalg.norm(x)
print(‘The value of a is:’, x,”\n\n”)
print(‘The value of norm1 is:’, norm1)
- Write a custom function to get unit vector:
- Approach 1:
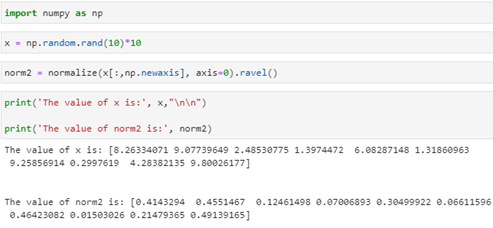
import numpy as np
x = np.random.rand(10)*10
norm2 = normalize(x[:,np.newaxis], axis=0).ravel()
print(‘The value of a is:’, x,”\n\n”)
print(‘The value of norm2 is:’, norm2)
- Approach 2:
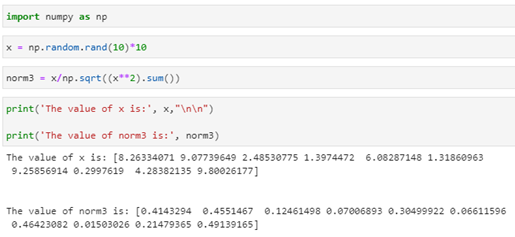
import numpy as np
x = np.random.rand(10)*10
norm2 = normalize(x[:,np.newaxis], axis=0).ravel()
print(‘The value of a is:’, x,”\n\n”)
print(‘The value of norm3 is:’, norm3)
Let’s see if values norm1, norm2 & norm3 are equal:

np.logical_and( (norm1==norm2).all(), (norm2==norm3).all() )
Summary
In this article, we learned about the different ways to convert a NumPy array into a unit vector. It can be done by using an internal function or by defining a user-defined function (UDF). The main reason behind calculating unit vector is when we care about direction rather than magnitude. This process of normalization involves removing magnitude.
References
Official documentation for linalg.norm library