SFTP (SSH File Transfer Protocol) is a secure file transfer protocol used for the management of encrypted data. SFTP is like FTP but with a security layer added when transferring data from the server to the client and vice versa. The program is run over a secure channel such as SSH, and the server has already verified the client. Thus, any web server following the SFTP protocol for the communication of data can be called an SFTP server. In this tutorial we shall learn how to go about connecting to an SFTP server.
For this, we require one SFTP server and a client-side application or library. There are many SFTP servers available publicly for testing, and we will be using one of them – “test.rebex.net”.
On client-side we will use the pysftp library in Python to connect with the targeted SFTP server. Pysftp is a high-level wrapper library based on the “paramiko” library in Python. Official documentation and release history of pysftp is available here.
Installation Of Pysftp
Make sure you have established an Internet connection and that pip is already installed on your system. Let’s then install pysftp:
- Open command line terminal
- Run command “pip install pysftp”
- Run Python
- Try to import pysfpt. If it succeeds means pysftp is now installed on your system
Installing pysftp results in adding some prerequisites libraries as shown below:

Python Module For SFTP Connection
Now that we have installed pysftp, let’s program it as follows:
# first of all import required libs.
import pysftp
host = 'test.rebex.net'
port = 22
username = 'demo'
password= 'password'
conn = pysftp.Connection(host=host,username=username, password=password)
In the above code snippet we have imported pydftp library in the first line. To initiate connection to the SFTP server, we need the server’s host address, port number (on which the SFTP server is running), username and password. We are using publicly available credentials for our targeted server (shown above). To explore more about this server go to this link.
We call pysftp.Connection() to create connection object named as conn, we pass host, username and password as parameters to pysftp.Connection() in last line of code.
Now save the above code snippet in file named, “sftp_client.py” and run it. As we run the SFTP client program for the first time on our system we get SSHException: Hostkey is required to trust targeted server.
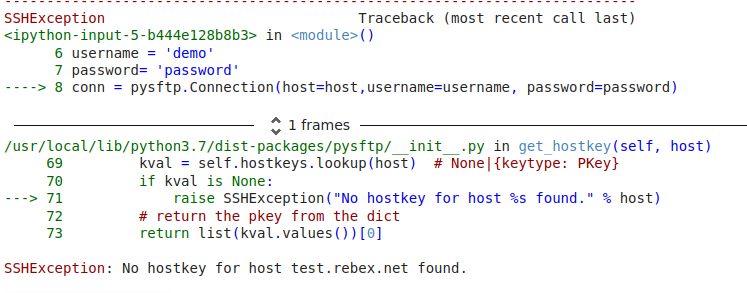
To handle the SSHException there are two options: either “skip to test host key for targeted server” or “add host key properly”.
Avoid Host Key Check
To “Avoid host key check” for targeted server “test.rebex.net”, open terminal and execute the following command:
“ssh -o StrictHostKeyChecking=no test.rebex.net”
It results in skipping host key checking for the server. A red flag here: skipping the host key check can result in a “man-in-the-middle” attack, so we will follow the correct method to add the host key.
How To Add Host Key Correctly
First, run the following command on your terminal to scan the publicly available SSH Key for targeted server:
ssh-keyscan ‘test.rebex.net’
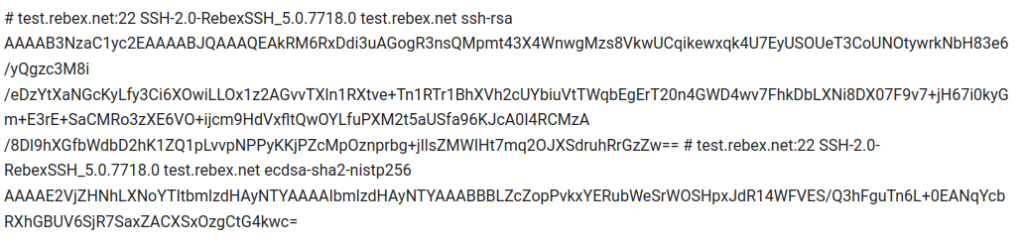
Now, run the following command to add this SSH key into SSH known hosts file:
ssh-keyscan -H ‘test.rebex.net’ >> ~/.ssh/known_hosts
Running the above command will print as shown below on the terminal. This means the host keys for targeted web-server are added successfully
# test.rebex.net:22 SSH-2.0-RebexSSH_5.0.7718.0
# test.rebex.net:22 SSH-2.0-RebexSSH_5.0.7718.0
# test.rebex.net:22 SSH-2.0-RebexSSH_5.0.7718.0
Let’s run the code again.
import time
import pysftp
import time
import pysftp
host = 'test.rebex.net'
port = 22
username = 'demo'
password= 'password'
try:
conn = pysftp.Connection(host=host,port=port,username=username, password=password)
print("connection established successfully")
except:
print('failed to establish connection to targeted server')
Save the above code snippet in sftp_client.py file and run.
Connection established successfully.
It will result in establishing a successful connection with SFTP server “test.rebex.net”.
Summary
In this article, we learned how to go about connecting to an SFTP server using the pysftp library in Python. We also learned about the challenges and the exceptions faced while using pysftp to establish a connection, and how to handle SSH host key exception by adding host key into .ssh/known hosts file.
In the next tutorial, we will show you the way to upload a file to an SFTP server using an established connection.