In the last tutorial we learned to make a successful connection with an SFTP server, to upload and download files, and to delete files on the targeted server using the pysftp client application. In this tutorial, we are going to learn about another package, Python “Paramiko”. We will explore how we can make a connection with the SFTP server using the Paramiko package, and what other operations are achievable.
Paramiko is a Python interface built around the SSHV2 protocol. By using Paramiko we can build client and server application as per the SSHV2 protocol requirements. It provides built-in functions which we can then use to create secure channels, and client/server applications easily.
Installation Of Python Paramiko
First, we have to make sure that Paramiko is installed in our system. The best way to install the latest release of Python Paramiko is to use the “pip” command in the following manner:
pip install paramiko
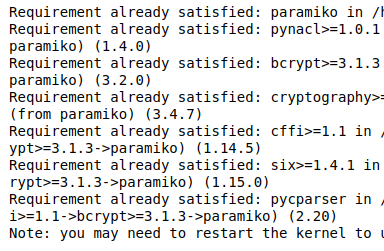
This will result in installing the required dependencies and Paramiko in a very short time. In case you are facing any problem using the above command, you can install Paramiko by passing the GitHub address of the package to the pip command in the following way:
pip install -e git+https://github.com/paramiko/paramiko/#egg=paramiko
SSH Client
We can use Secure Shell Protocol, SSHClient() method of Paramiko package to create a SSH client in our program. SSH clients are used to communicate with an SFTP server for the transfer of files.
So, we import our installed package Paramiko and create the SSH client in the following manner:
# import required packages
import paramiko
# create ssh client
ssh_client = paramiko.SSHClient()
Now we define the parameter of our targeted server.
server = 'test.rebex.net'
port = 22
user = 'demo'
password = 'password'
Now that we have the credentials of our targeted server, and we have also created an SSH client using SSHClient() method of Paramiko, let’s go ahead and connect with the server now.
# import required packages
import paramiko
# create ssh client
ssh_client = paramiko.SSHClient()
# declare credentials of targeted server
server = 'test.rebex.net'
port = 22
user = 'demo'
password = 'password'
# establish connection with targeted server
ssh_client.connect(server,port,user,password)
If we run the above command we get the following error:
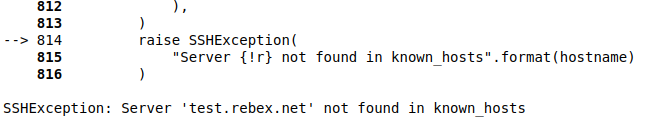
The error clearly states that we have not added “test.rebex.net” as “know_host” to our machine. It means our computer does not trust our targeted server test.rebex.net. To handle this, Python Paramiko provides a mechanism to add the policy, “trusting server or rejecting it”. By default, Paramiko takes it as rejection policy as can be seen in the above code. But if we call ssh_client.set_missing_host_key_policy(), and we pass “paramiko.AutoAddPolicy()” as argument, then Paramiko will make our machine to trust incoming traffic from our server test.rebex.net.
Let’s call ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) as following:
# import required packages
import paramiko
# create ssh client
ssh_client = paramiko.SSHClient()
server = 'test.rebex.net'
port = 22
user = 'demo'
password = 'password'
ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh_client.connect(server,port,user,password)
print('connection established successfully')
So far we have been able to establish the connection to our server test.rebex.net successfully. Now we will run exec_command() of ssh_client to get the secure channel easily.
Executing Commands On Server
Using Paramiko’s SSH client we can execute commands on our targeted server. Let’s say we want to list down the files and directories in the root folder on test.rebex.net. We can do it in the following manner:
We will use SSHClient.exec_command() to run the command on server. We will pass “ls” as command to exec_command() method.
stdin,stdout,stderror=ssh_client.exec_command('ls')
exec_command(‘ls’) returns three channels. First, channel stdin is used if we want to pass the input as a parameter to command. stdout is used to get the result, and stderror is used to deal with the errors.
stdout.read_lines()

So we can see that stout.readlines() prints the names of the files and the folders in the directory.
Summary
In this tutorial, we explored the Paramiko package in Python, built around the SSHV2 protocol. We learned to install and create the SSH client and also established a connection with the testing SFTP server. We also explored how to add any server to known hosts using Paramiko’s AutoAddPlolicy(). We saw that Paramiko provides the right channel to establish the connection with the SFTP server, and we can execute commands on the targeted server as well.
References
Recommended Books:
- Mastering Python for Networking and Security
- Effective Python: 90 Specific Ways to Write Better Python
The books above are affiliate links which benefit this site.