When it comes to communicating with an SFTP server to exchange files, sometimes we have to delete the remote files on the server. Our pysftp library provides a proper mechanism to remove a particular file from the SFTP server. While deleting the files from the server we need an established connection to the SFTP server, the path of the targeted file to be deleted, and the permission from the server to delete the designated file. So, as you may have gathered, in this tutorial we shall learn all about deleting files on SFTP server.
Let’s establish a connection with the targeted server, “test.rebex.net”.
import pysftp
host = 'test.rebex.net'
port = 22
username = 'demo'
password= 'password'
try:
conn = pysftp.Connection(host=host,port=port,username=username, password=password)
print("connection established successfully")
except:
print('failed to establish connection to targeted server')
connection established successfully
Validating Existence Of File
After establishing a connection to the SFTP server, we will validate if the file exists in the given directory path. We will be using conn.exists() method to validate if the file exists or not. In this case, our targeted file is a “ResumableTransfer.png” in ‘/pub/example’ directory of the SFTP server ‘test.rebex.net’.
with conn.cd('/pub/example'):
if(conn.exists('ResumableTransfer.png')):
print('file exists')
file exists
Now, let’s try and remove ResumableTransfer.png by doing the following:
with conn.cd('/pub/example'):
if(conn.exists('ResumableTransfer.png')):
print('file exists')
try:
print('remving file')
conn.remove('ResumableTransfer.png')
except Exception as e:
print(e)
file exists
removing file
[Errno 13] Access denied
So here we put conn.remove(file) in try catch block. We can see that our code has thrown an exception that tells us that we don’t have proper permission from the server to delete the said file. As we know that test.rebex.net is a test public server, it will not allow us to modify the files on it from a public account.
To test our code, we will have to modify our program to first connect with the SFTP server running on a local machine, and then try and remove the file there.
Connect To Local SFTP Server
Continuing with this lesson on deleting files on SFTP server; we will now establish a connection with the local SFTP server running on our local machine in the following way:
import pysftp
# credentials of targeted sftp server
host = '127.0.0.1'
port = 22
username = 'myuser'
password= 'mypassword'
try:
conn = pysftp.Connection(host=host,port=port,username=username,password=password)
print("connection established successfully")
except:
print('failed to establish connection to targeted server')
connection established successfully
Now, we want to remove all “txt” extension files inside the temp-files directory.
conn.listdir()
It prints all available files inside the current working directory, and we want to remove all txt files, so first we list down all txt files and then run the command to delete all these files in the following way:
with conn.cd('/tmp-files/'):
files = conn.listdir()
for file in files:
if (file[-4:]=='.txt'):
conn.remove(file)
print(file,' deleted successfully ')
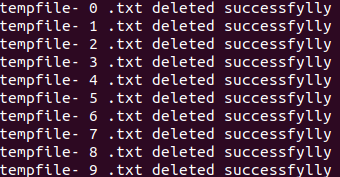
So, now you can see that all the files with the txt extension have been deleted successfully.
Summary
In this tutorial we learned how to delete files on an SFTP server using the pysftp library in Python. The test.rebex.net server does not give the permission to delete the files, so what we had to do was to establish a connection to the SFTP server running on a local computer. Then, we tried to delete the files using pysftp. We also understood from this tutorial that we need to validate the file path on the server, and that we need proper permission from the SFTP server before running the delete operation on that server.