In this tutorial you will learn how to download files from an SFTP server. This lesson is in continuation of our previous tutorial, “Connecting SFTP Server In Python” where you had learned to establish a connection with the SFTP server by adding proper SSH keys on the client-side machine for the targeted SFTP server.
So, getting on with this lesson: Let’s look at the code snippet where we are using the “Pysftp Library” to establish a connection with the targeted server, “test.rebex.net”.
import pysftp
host = 'test.rebex.net'
port = 22
username = 'demo'
password= 'password'
try:
conn = pysftp.Connection(host=host,port=port,username=username, password=password)
print("connection established successfully")
except:
print('failed to establish connection to targeted server')
Running the above code results in the following output:
“….connection established successfully”
Getting File From Established Connection To SFTP Server
In the code there’s a variable named “conn” which is created using the pysftp.Connection() method. We will call the get() method of conn object to get the targeted file. But first, we have to get into the targeted directory.
Check the current working directory for our conn object using the cwd method.
import pysftp
host = 'test.rebex.net'
port = 22
username = 'demo'
password= 'password'
try:
conn = pysftp.Connection(host=host,port=port,username=username, password=password)
print("connection established successfully")
except:
print('failed to establish connection to targeted server')
current_dir = conn.pwd
print('our current working directory is: ',current_dir)

So you are in the root directory now. Let’s check the available list of directories. To get the list, we will call listdir() method of conn object as following:
print('available list of directories: ',conn.listdir())
Let’s download the ‘readme.txt’ file.
conn.get(‘readme.txt’)
If you run the above command, nothing will be printed, but if you check your directory on a local machine, a new file readme.txt would have been downloaded there.
Moving To Specific Directory And Downloading File
So far we have downloaded a single file residing in the server’s root directory. What if we were given a path of the directory, and we had to download the file from that directory? After all, this tutorial is to help you understand how to download files from SFTP server.
Our targeted path is: “/pub/example”, and we want to download, “ResumableTransfer.png”.
conn.get('/pub/example/ResumableTransfer.png')
When we run the above code, it results in the downloading of ResumableTransfer.png into the local machine as shown below:
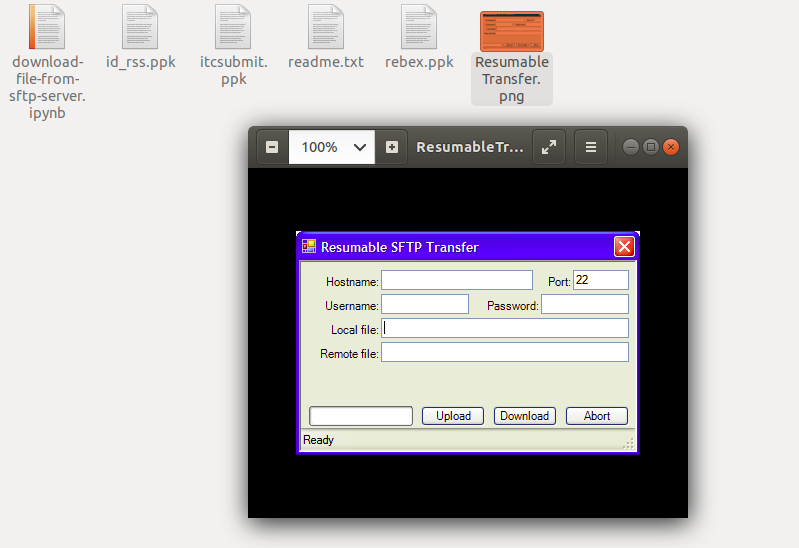
An Alternate Approach
So far we have passed the complete path to our conn.get() method, which results in the downloading of the targeted file. Now, let’s try another approach as shown below:
with conn.cd('pub/example/'):
conn.get('ResumableTransfer.png')
Here, we first move to the targeted directory using the conn.cd() method. Then, we just pass the file name to the conn.get() method. This leads to the downloading of that file into the local directory of our client-side local machine.
How To Download Multiple Files For Given Extension
There are cases when we have to download all the files in a directory with the given extension type. Let’s say, you want to download all the png files available in the “/pub/example” directory on the test.rebex.net server. Here’s how it is done:
with conn.cd('pub/example/'):
files = conn.listdir()
for file in files:
if (file[-4:]=='.png'):
conn.get(file)
print(file,' downloaded successfully ')
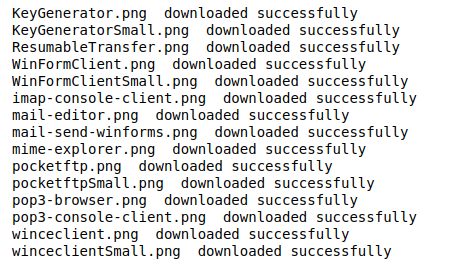
In the above code snippet we have moved into the specific directory using the conn.cd() method. We then got the list of files available in that directory using the conn.listdir() method. Following that, we iterated each file and checked if it was a png file that was downloaded using the conn.get() method or not.
Summary
In this tutorial, we have learned how to download files from an STFP server using the pysftp package in Python. To begin with, we tried to establish the connection with the server. Once successful, we tried two multiple approaches to download the required files. We learned how to print the current working directory using the pwd method. We also understood how to move to a specific directory, and download all such files having a specific extension.
References
Recommended Books:
- Mastering Python for Networking and Security
- Effective Python: 90 Specific Ways to Write Better Python
The books above are affiliate links which benefit this site.