Azure is a cloud storage service provided by Microsoft. Azure provides Python API “Azure” to enable Python programmers to interact with cloud storage easily. In this tutorial we shall learn to use Azure Python SDK to move files from a local machine to the Azure cloud storage.
Requirements
For the purposes of this tutorial, you must have Azure installed in your Python environment. You can install the Azure SDK using pip in the following manner:
pip install azure
Further, you must have an Azure account with cloud storage as an active service on it. After all, this tutorial is about understanding how to use Azure Python SDK to move files to the Azure cloud storage. To learn about how to create the Azure account and activate cloud storage, please click here.
Let’s create our first project on the Azure cloud storage named, “myfirstproject”. Under that, we create a container named, “datacourse-007”. When you create the new account on Azure, it provides you a portal, which you can use to create a project and also create a container in it as shown below:
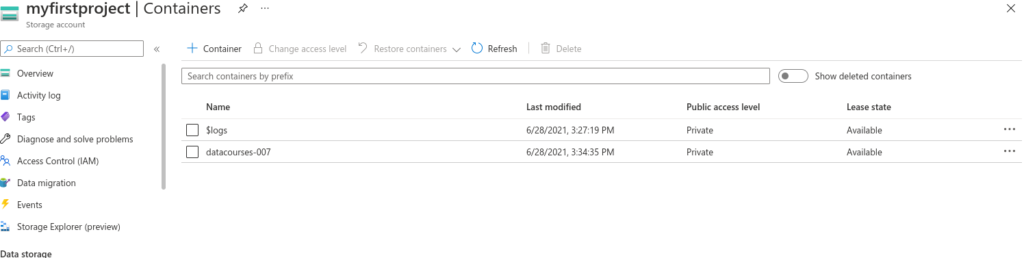
So far we have installed the Azure Python Library using pip, and we have set up the container on the cloud storage account.
Let’s move forward to our Python programming part.
Upload File To Azure Storage
When we create a container, Azure provides you a connection string as a secret key, which you can use to access cloud storage from third party apps (in this case our Python program).
So let’s first import the Azure library in Python and store that connection string in conn_string variable as shown below:
from azure.storage.blob import BlobClient
# paste connection string below
conn_str = "****************************"
On Azure storage, files are treated as Blobs. So when you upload any file to Azure, it will be referred to as a Blob.
To upload a file as a Blob to Azure, we need to create BlobClient using the Azure library.
blob_client = BlobClient(conn_string=conn_str,container_name="datacourses-007",blob_name="testing.txt")
While creating blob_client, you must pass connection_string, name of container and blob_name as parameter to BlobClient() method.
Once blob_client is created we can open the stream to our file and can call blob_client.upload_data() method to upload content of that file to Azure Blob.
# let's upload file to blob
try:
with open('testing.txt',"rb") as f:
blob.upload_blob(f)
print('testing.txt uploaded to container: datacourses-007 successfully')
except Exception as e:
print(e)

Let’s verify it by refreshing our container portal on Azure.
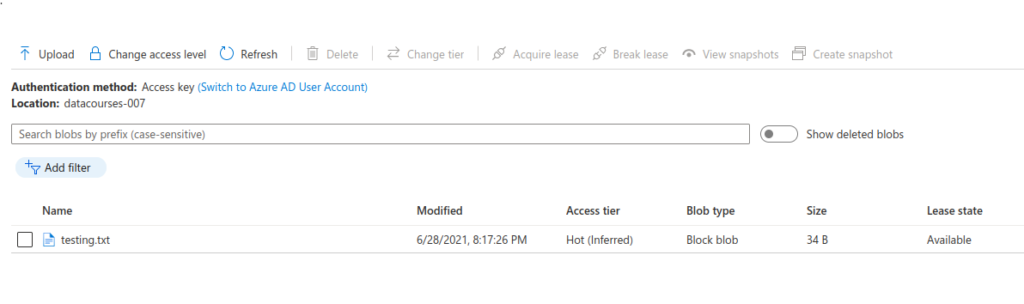
So here we can see that our testing.txt file is uploaded as a Blob to our container, datacourses-007.
Download File From Azure Container
Now, we write a program to download a file from the Azure container.
First of all, we import BlobClient from azure.storage.blob and pass our connection_string to variable conn_str.
from azure.storage.blob import BlobClient
# enter your connection string below
conn_str = "**************************"
Now we initiate blob object as following:
blob = BlobClient.from_connection_string(conn_str=conn_str,container_name="datacourses-007", blob_name="testing.txt")
Open file writer stream with name of file “downloaded_testing.txt” and call blob.download_blob() method.
try:
with open('downloaded_testing.txt',"wb") as f:
f.write(blob.download_blob().readall())
print('testing.txt downloaded from container: datacourses-007 successfully')
except Exception as e:
print(e)
testing.txt downloaded from container: datacourses-007 successfully
Summary
In this tutorial we learned to upload and download files from Azure cloud storage containers. We explored methods to upload files and download files using the Python API provided by Azure. We understood about structure of Azure cloud storage, containers and Blobs.